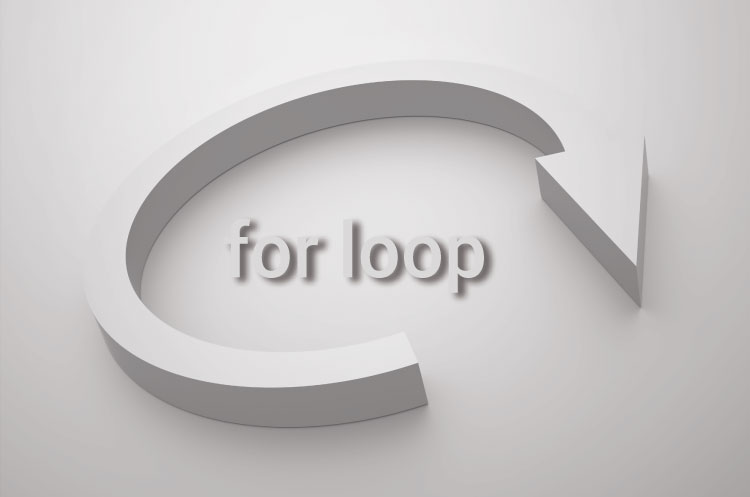
Loops are used in programming to repeat a specific block until some end condition is met. There are three type of loops in C++ programming:
- for loop
- while loop
- do...while loop
C++ for Loop Syntax
for(initializationStatement; testExpression; updateStatement) { // codes }
where, only testExpression is mandatory.
How for loop works?
- The initialization statement is executed only once at the beginning.
- Then, the test expression is evaluated.
- If the test expression is false, for loop is terminated. But if the test expression is true, codes inside body of
for
loop is executed and update expression is updated. - Again, the test expression is evaluated and this process repeats until the test expression is false.
Flowchart of for Loop in C++
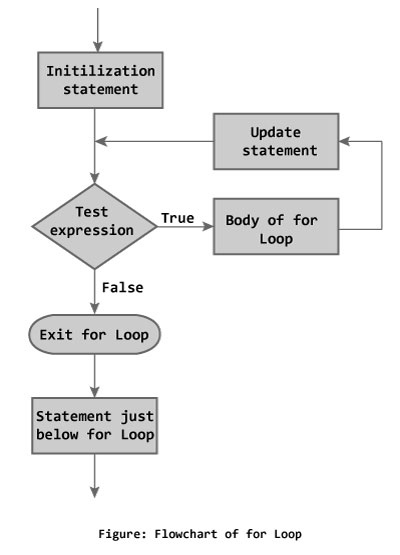
Example 1: C++ for Loop
// C++ Program to find factorial of a number
// Factorial on n = 1*2*3*...*n
#include <iostream>
using namespace std;
int main()
{
int i, n, factorial = 1;
cout << "Enter a positive integer: ";
cin >> n;
for (i = 1; i <= n; ++i) {
factorial *= i; // factorial = factorial * i;
}
cout<< "Factorial of "<<n<<" = "<<factorial;
return 0;
}
Output
Enter a positive integer: 5 Factorial of 5 = 120
In the program, user is asked to enter a positive integer which is stored in variable n(suppose user entered 5). Here is the working of
for
loop:- Initially, i is equal to 1, test expression is true, factorial becomes 1.
- i is updated to 2, test expression is true, factorial becomes 2.
- i is updated to 3, test expression is true, factorial becomes 6.
- i is updated to 4, test expression is true, factorial becomes 24.
- i is updated to 5, test expression is true, factorial becomes 120.
- i is updated to 6, test expression is false,
for
loop is terminated.
In the above program, variable i is not used outside of the
for
loop. In such cases, it is better to declare the variable in for loop (at initialization statement).#include <iostream>
using namespace std;
int main()
{
int n, factorial = 1;
cout << "Enter a positive integer: ";
cin >> n;
for (int i = 1; i <= n; ++i) {
factorial *= i; // factorial = factorial * i;
}
cout<< "Factorial of "<<n<<" = "<<factorial;
return 0;
}